spring learning code 1
This is my learning note about how Spring core features like IoC, AOP works, with Code Examples .
Spring Code Learning Notes with Code Examples
Table of Contents
- Spring Code Learning Notes with Code Examples
- Table of Contents
- spring introduce
- Spring IOC
- <em><strong>1. spring ioc basic concepts</strong></em>
- <em><strong>2. IOC interfaceBeanFactory</strong></em>
- <em><strong>3. IOC opertation Bean managementxml</strong></em>
- <em><strong>4. Bean managementxml external beans</strong></em>
- <em><strong>5. Bean managementxml inner beans and Cascade assignment</strong></em>
- <em><strong>6. Bean managementxml array, list, map</strong></em>
- <em><strong>7. Bean managementset object type in collection</strong></em>
- <em><strong>8. extract injection in collection</strong></em>
- <em><strong>9. IOC manage Bean factory beans</strong></em>
- <em><strong>10. Spring Bean Scopes</strong></em>
- <em><strong>11. Spring Bean Lifecycle</strong></em>
- <em><strong>12.bean post processor 7 steps of life cycle</strong></em>
- <em><strong>13.ioc 管理 bean (xml autowire)</strong></em>
- <em><strong>14.ioc 管理 bean (外部属性)</strong></em>
spring introduce
download
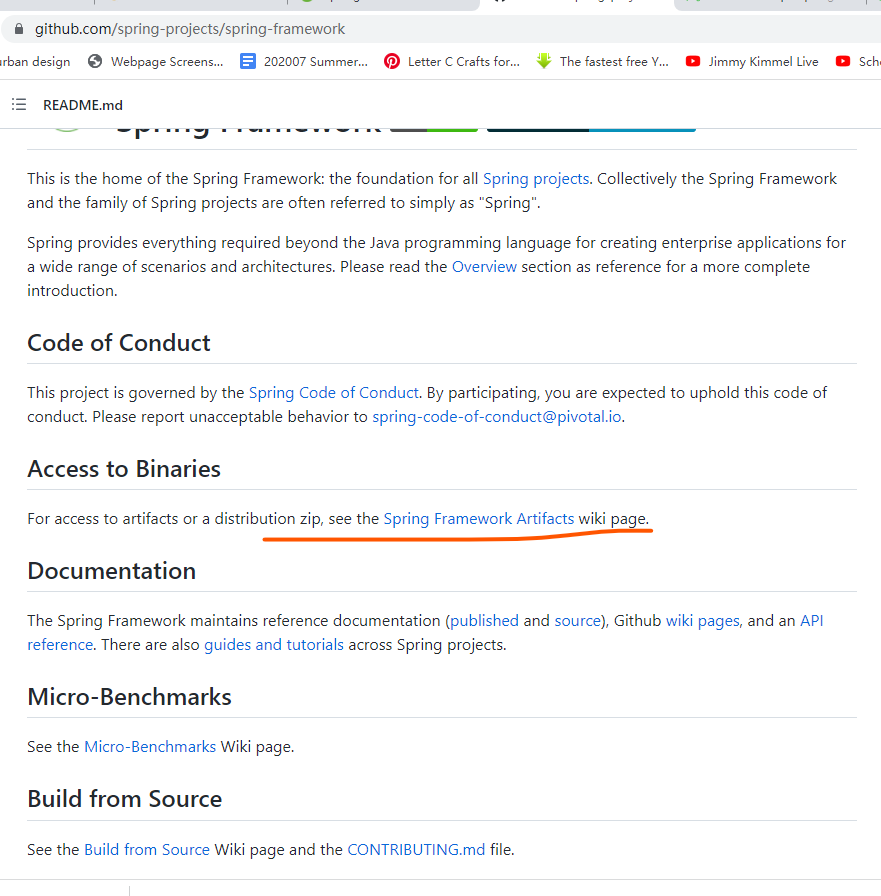
spring
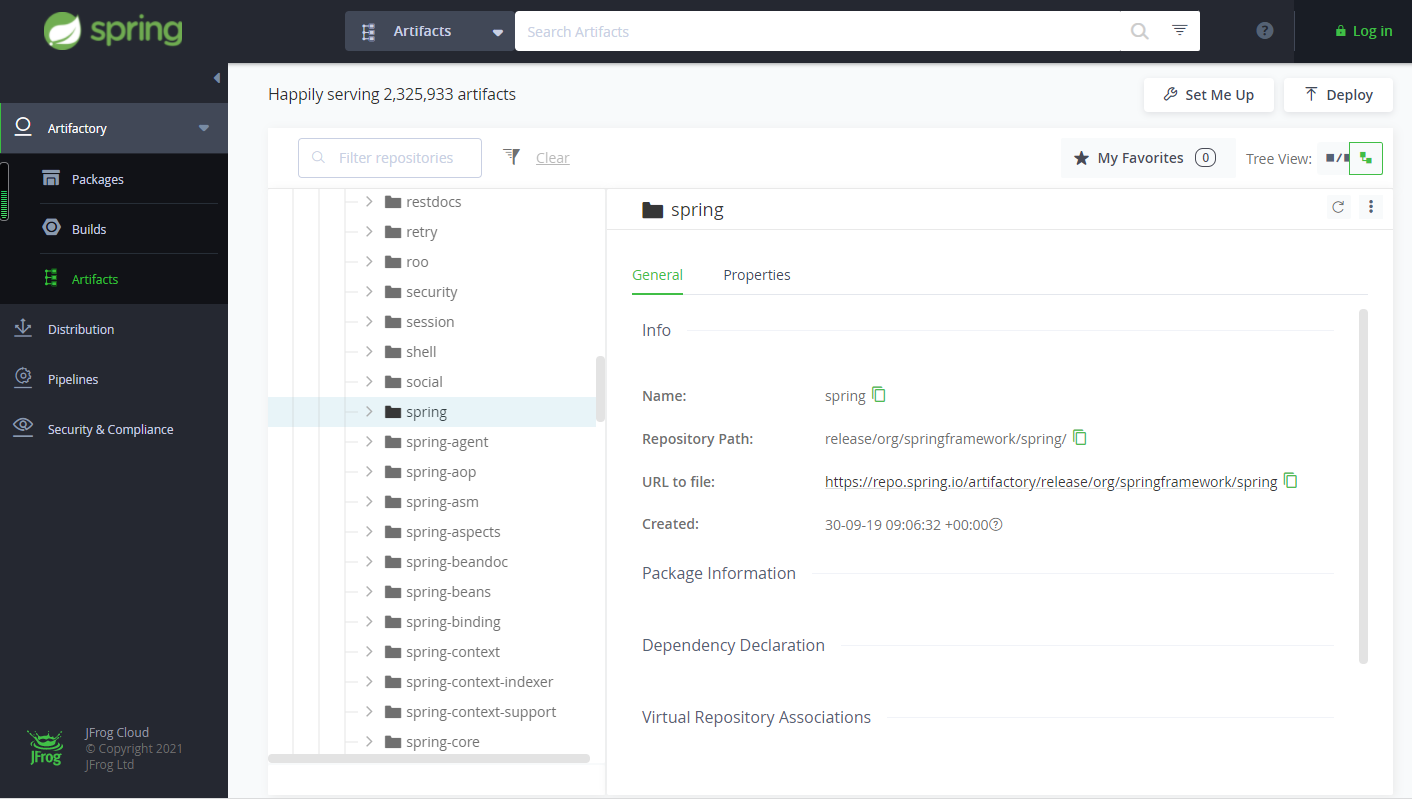
spring
inport jar
-
Beans
-
Core
-
Context
-
Expression
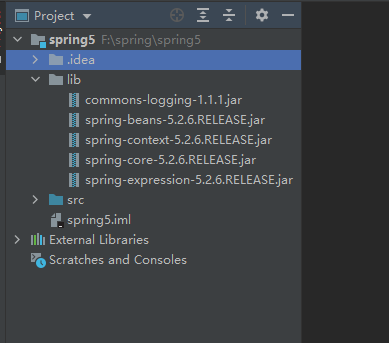
spring
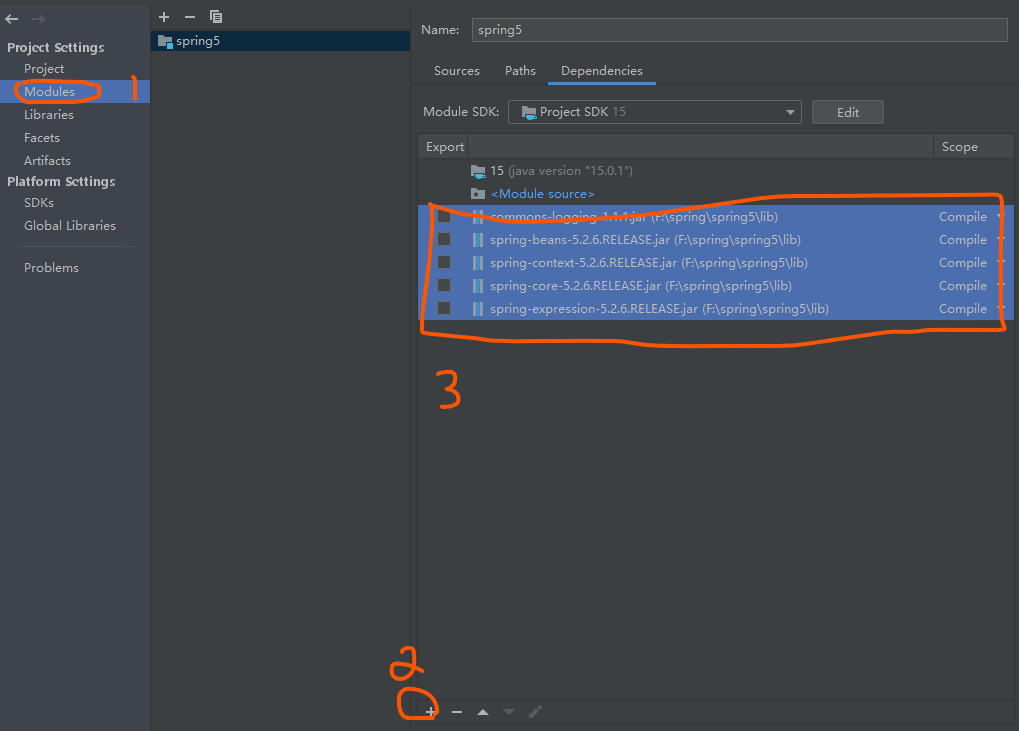
spring jar
create class and mothod
public class User {
public void add() {
System.out.println("add.............");
}
}
create an object with Spring properties
1. xml file in Spring properties
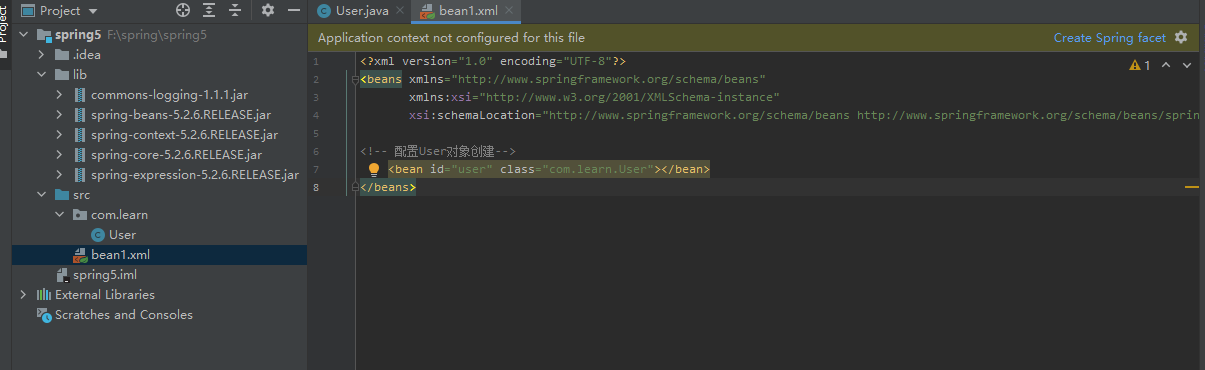
spring jar
test
1. load spring properties file
2. get object from properties (bean id)
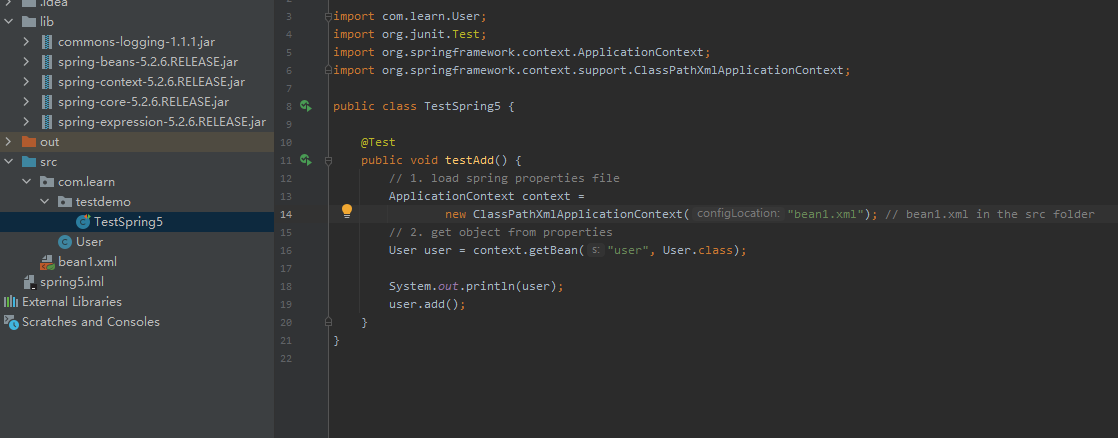
spring jar
Spring IOC
1. spring ioc basic concepts
-
xml encoding, factory-based design pattern, reflect
-
original: tightly coupled
class UserService{
execute();
UserDao dao = new UserDao();
dao.add();
}
class UserDao{
add() {
...
}
}
- factory-based design pattern
class UserService{
execute();
UserDao dao = UserFactory.getDao();
dao.add();
}
class UserDao{
add() {
...
}
}
class UserFactory{
public static UserDao getDao() {
return new UserDao();
}
}
- IOC
<bean id="user" class="com.learn.User"></bean>
class UserFactory{
public static UserDao getDao() {
// 1. xml encoding
String classValue = class属性;
// 2. use reflect create an object
Class clazz = Class.for(Name);
return (UserDao)clazz.newInstance();
}
}
2. IOC interface(BeanFactory)
-
BeanFactory: for String, not for developer
- won’t creates object when loading properties file
-
ApplicationContext
- creating objects when loading properties file
3. IOC opertation Bean management(xml)
- creating objects in xml(bean)
-
bean tag properties
- id, class…
- create objects by No-argument constructor
-
set properties in xml
- DI: set properties
-
DI: set method
- 1). create class, and set method
public void setBname(String bname) {
this.bname = bname;
}
- 2). spring xml file, set properties
- DI: use annotation
- 1). create class, and set method, and argument constructor
public class Orders{
private String oname;
private String address;
public Order(String oname, String address) {
this.oname = oname;
this.address = address;
}
}
- 2). spring xml file, use argument constructor
4. Bean management(xml) external beans
- 2 classes: service and dao
- call methods in dao from service class
// service and dao objects: 在service层创建UserDAO类,生成set方法
<bean id="userService" class="com.learn.service.UserService">
// inject userDao object
// name attribute: class attribute name private UserDao userDao;
<property name="userDao" ref="userDaoImpl"></property>
</bean>
<bean id="userDaoImpl" class="com.learn.dao.UserDaoImpl"></bean>
5. Bean management(xml) inner beans and Cascade assignment
- 1 对多关系: 部门和员工
- 在实体类之间表示 1 对多关系
department
public class Dept {
private String dname;
public void setDname(String dname) {
this.dname = dname;
}
}
employ
public class Emp {
private String ename;
private Dept dept;
public void setDept(Dept dept) {
this.dept = dept;
}
}
xml
// inner bean
<bean id="emp" class="com.learn.bean.Emp">
// inject userDao object
<property name="ename" value="lucy"></property>
// method 1
// <property name="dept" ref="dame"></property>
// method 2
<property name="dept">
<bean id="dept" class="com.learn.bean.Dept">
<property name="dname" value="IT"></property>
</property>
</bean>
6. Bean management(xml) array, list, map
6.1 class and set method
public class Stu {
// array
private String[] courses;
// list
private List<String> list;
// map
private Map<String, String> maps;
// set
private Set<String> sets;
public void setCourses(String[] courses) {
this.courses = courses;
}
public void setList( List<String> list) {
this.list = list;
}
public void setMap(Map<String, String> maps) {
this.maps = maps;
}
public void setSet(Set<String> sets) {
this.sets = sets;
}
}
6.2 xml
<bean id="stu" class="com.learn.conllectiontype.Stu">
// array
<property name="courses">
<array>
<value>java class </value>
<value>sql class </value>
</array>
</property>
// list
<property name="list">
<list>
<value>AAA </value>
<value>BBB class </value>
</list>
</property>
// MAP
<property name="maps">
<map>
<entry>key="JAVA" value="jva"</entry>
<entry>key="PHP" value="php"</entry>
</map>
</property>
// set
<property name="sets">
<set>
<value>MySQL </value>
<value>Redis </value>
</set>
</property>
</bean>
7. Bean managementset object type in collection
7.1 class and set method
public class Course {
private String cname;
public void setCname(String cname) {
this.cname = cname;
}
public String toString() {
}
}
public class Stu {
// array
private String[] courses;
// list
private List<String> list;
// map
private Map<String, String> maps;
// set
private Set<String> sets;
// ===============coursed students take===============
private List<Course> courseList;
public void setCourseList(List<Course> courseList) {
this.courseList = courseList;
}
// ===============coursed students take===============
public void setCourses(String[] courses) {
this.courses = courses;
}
public void setList( List<String> list) {
this.list = list;
}
public void setMap(Map<String, String> maps) {
this.maps = maps;
}
public void setSet(Set<String> sets) {
this.sets = sets;
}
}
7.2 xml
<bean id="stu" class="com.learn.conllectiontype.Stu">
// array
<property name="courses">
<array>
<value>java class </value>
<value>sql class </value>
</array>
</property>
// list
<property name="list">
<list>
<value>AAA </value>
<value>BBB class </value>
</list>
</property>
// MAP
<property name="maps">
<map>
<entry>key="JAVA" value="jva"</entry>
<entry>key="PHP" value="php"</entry>
</map>
</property>
// set
<property name="sets">
<set>
<value>MySQL </value>
<value>Redis </value>
</set>
</property>
</bean>
// ===============inject list collection type, value is object===============
<property name="courseList">
<list>
<ref bean="course1"></ref>
<ref bean="course2"></ref>
</list>
</property>
// ===============create multiple course objects===============
<bean id="course1" class="com.learn.collectiontype.Course">
<property name="cname" value="Spring5"></property>
</bean>
<bean id="course2" class="com.learn.collectiontype.Course">
<property name="cname" value="MyBatis"></property>
</bean>
8. extract injection in collection
public class Book {
private List<String> list;
public void setList(List<String> list) {
this.list = list;
}
public String toString() {
}
public void test() {
System.out.println(list);
}
}
8.2 in xml import name space util
<bean xmlns=""
xmlns:p=""
// copy xmlns:p
xmlns:util="http://www.springframework.org/schema/util"
// change xsi:schemaLocation beans to util
// xsi:schemaLocation="http://www.springframework.org/schema/beans" "http://www.springframework.org/schema/beans/spring-beans.xsd"
xsi:schemaLocation="http://www.springframework.org/schema/util" "http://www.springframework.org/schema/util/spring-util.xsd">
8.3 util tag
// 1. list=====================
<util:list id="bookList">
<value>AAA </value>
<value>BBB</value>
<value>CCC</value>
</util:list>
8.4 test
@Test
public void testCollection() {
ApplicationContext context = new ClassPathXmlApplicationContext("bean2.xml");
Book book = context.getBean("book", Book.class);
book.test();
}
9. IOC manage Bean (factory beans)
9.1 ordinary beans 定义 bean 类型就是返回类型
9.2 factory beans 配置文件定义 bean 类型和返回类型不一样
-
9.2.1 创建类,作为工厂 bean, 实现接口 FactoryBean
-
9.2.2 实现接口里面的方法,在方法中定义返回的 bean 类型
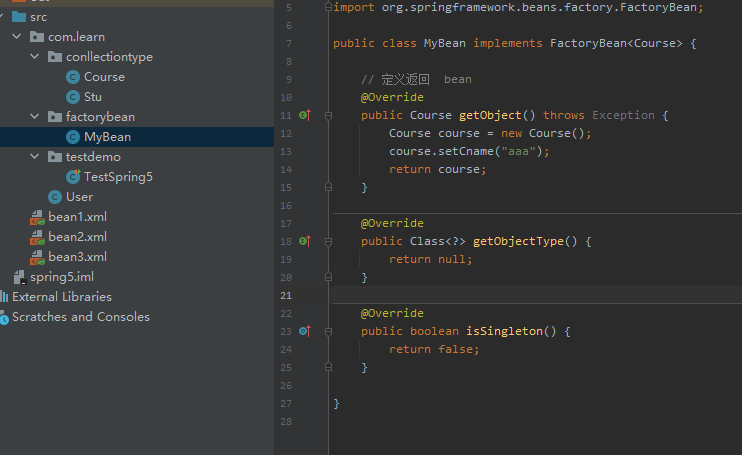
spring factorybean
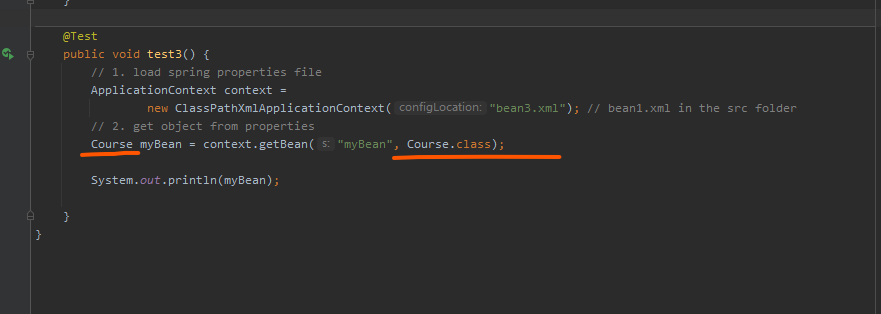
spring factorybean
10. Spring Bean Scopes
10.1 single or instances will be created 单实例多实例 10.2 default model is single instance 默认 spring bean 是单实例对象 10.3 scope is used to set single or multiple: singleton or protype 10.4 singleton vs protype
- singleton: create instance when load spring xml file
- prototype: create instances when getBean method
11. Spring Bean Lifecycle
11.1 create instance by constructor(no-argument constructor)
Spring Bean Lifecycle
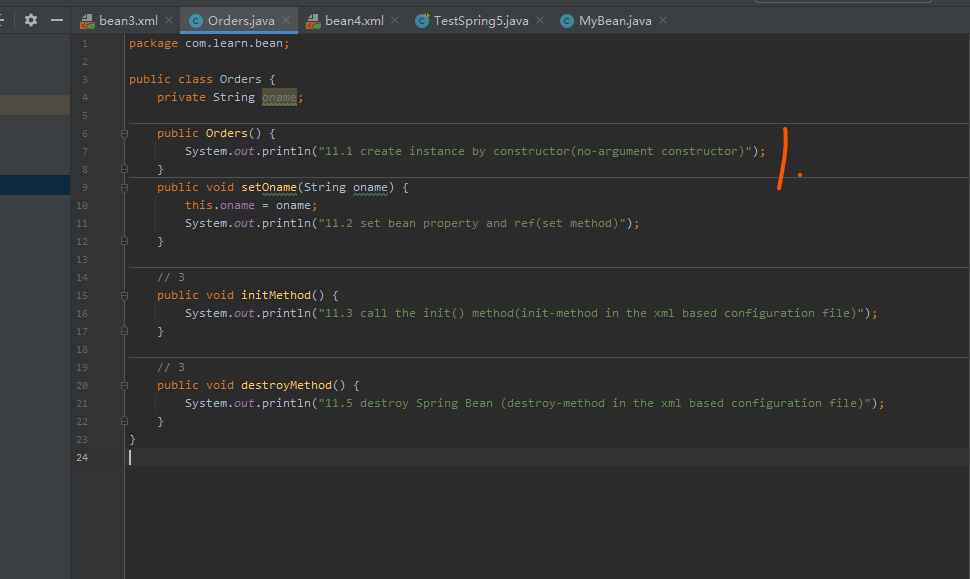
Spring Bean Lifecycle
11.2 set bean property and ref(set method)
Spring Bean Lifecycle
11.3 call the init() method(init-method in the xml based configuration file)
Spring Bean Lifecycle
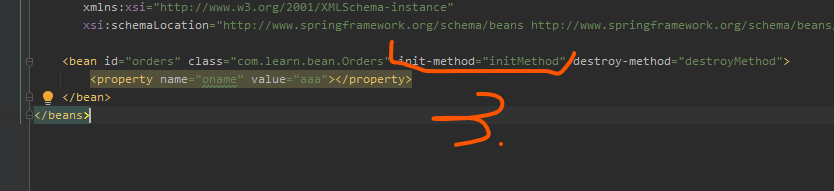
Spring Bean Lifecycle
11.4 use bean
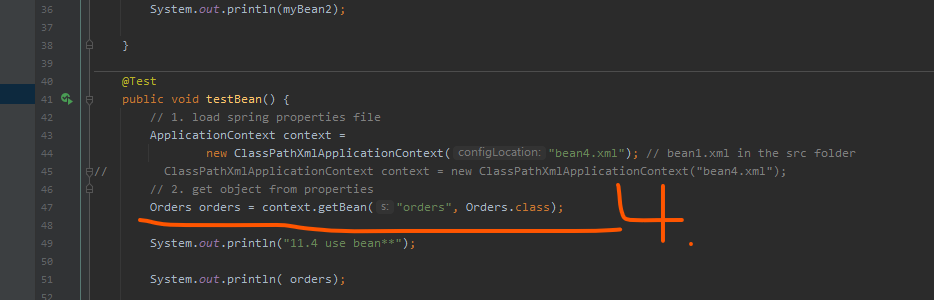
Spring Bean Lifecycle
11.5 destroy Spring Bean (destroy-method in the xml based configuration file)
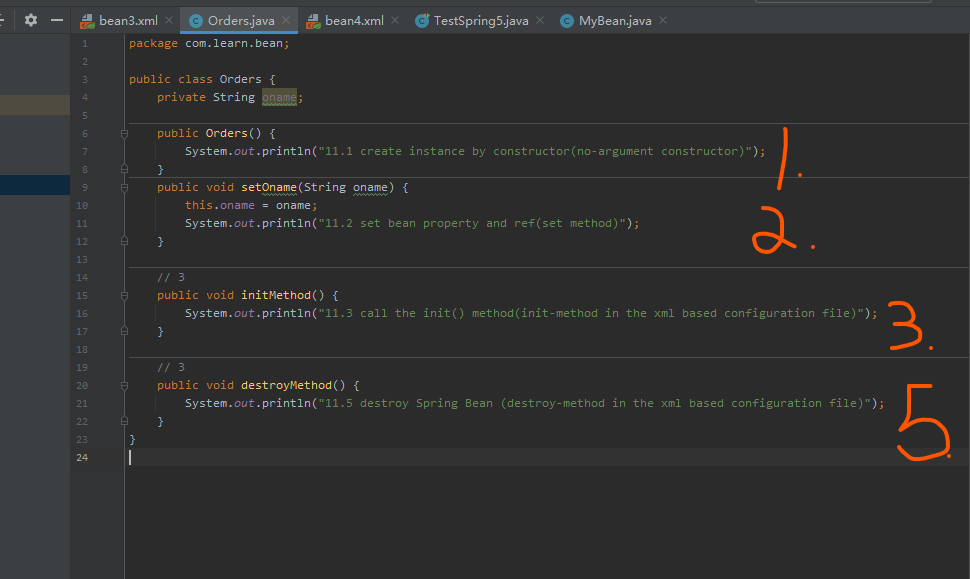
Spring Bean Lifecycle
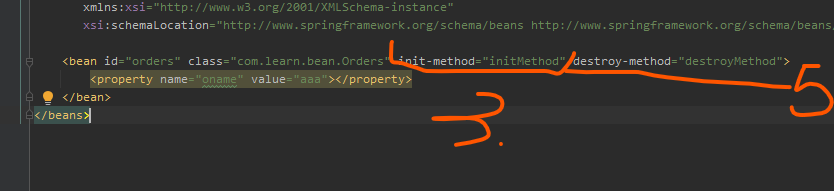
Spring Bean Lifecycle
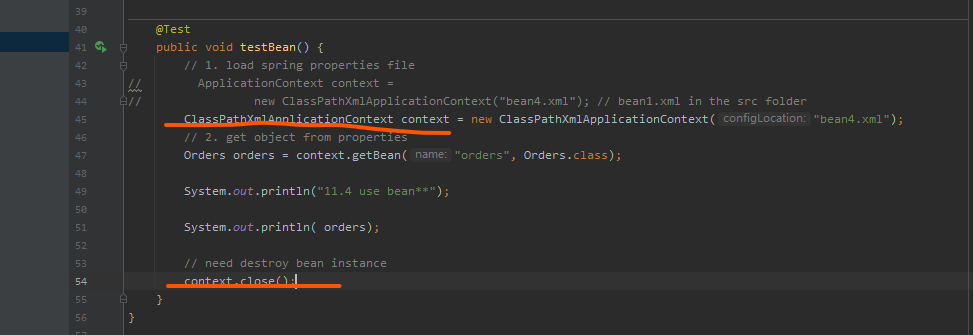
Spring Bean Lifecycle
12.bean post processor (7 steps of life cycle)
12.1 create instance by constructor(no-argument constructor)
- create class, implement interface BeanPostProcessor
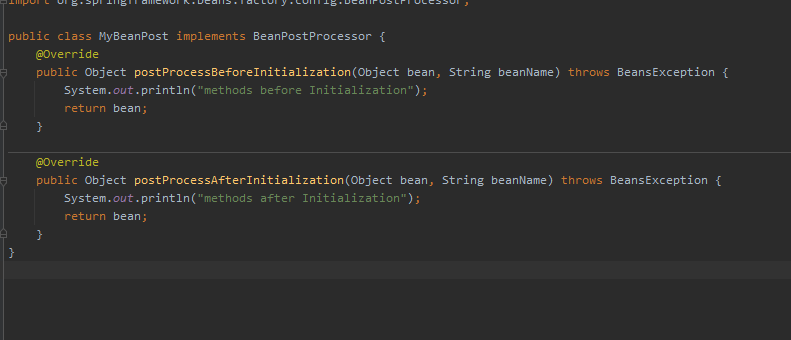
Spring Bean Lifecycle
12.2 set bean property and ref(set method)
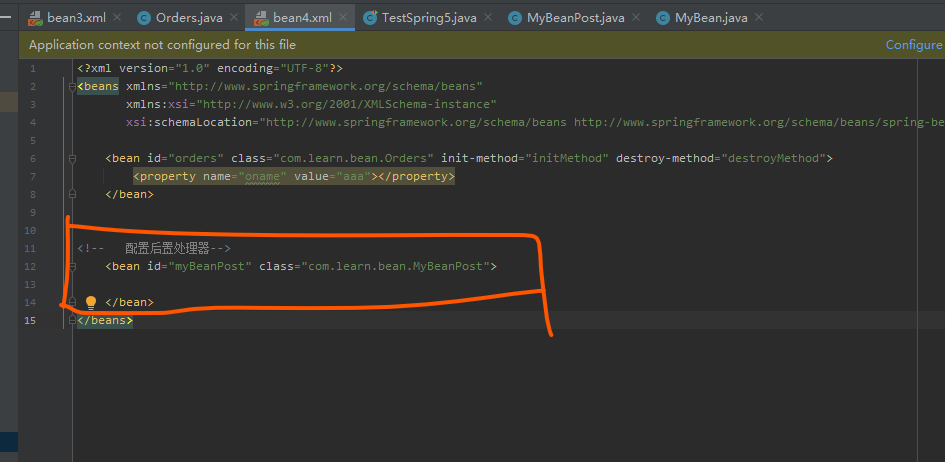
Spring Bean Lifecycle
12.3 pass bean instance to post processor
12.4 call the init() method(init-method in the xml based configuration file)
12.5 pass bean instance to post processor
12.6 use bean
12.7 destroy Spring Bean (destroy-method in the xml based configuration file)
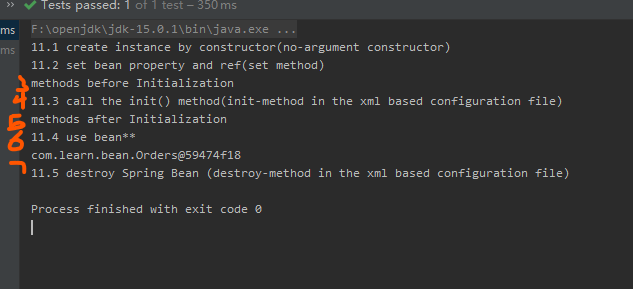
Spring Bean Lifecycle
13.ioc 管理 bean (xml autowire)
13.1 autowire: byName: 注入值 bean 的 id 值和类里面属性名称要一样
13.2 autowire: byName, byType
14.ioc 管理 bean (外部属性)
14.1 直接配置数据库信息(not recommend)
Spring ioc
14.2 引入外部属性文件配置数据库连接池
create properties file
Spring ioc
import context name space
Spring ioc
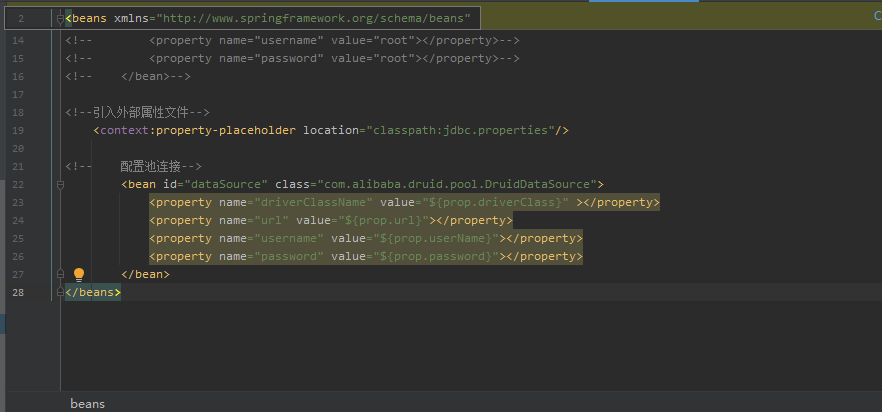