Builder, Prototype design pattern
1. Builder: design a DecorationPackage
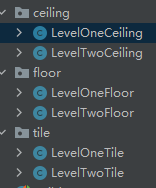
Builder
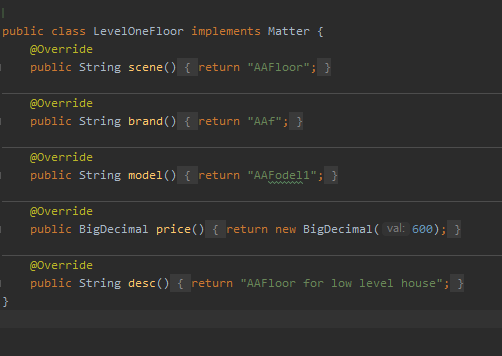
Builder
public interface Imenu {
Imenu appendCeiling(Matter matter);
Imenu appendFloor(Matter matter);
Imenu appendTile(Matter matter);
String getDetail();
}
Matter => (interface)
- ceiling
- LevelOneCeiling
- LevelTwoCeiling
- floor
- LevelOneFloor
- LevelTwoFloor
- tile
- LevelOneTile
- LevelTwoTile
IMenu => IMenu method(Matter matter)
-
Imenu appendCeiling(Matter matter);
-
Imenu appendFloor(Matter matter);
-
Imenu appendTile(Matter matter);
-
String getDetail();
IMenuImpl => Deco
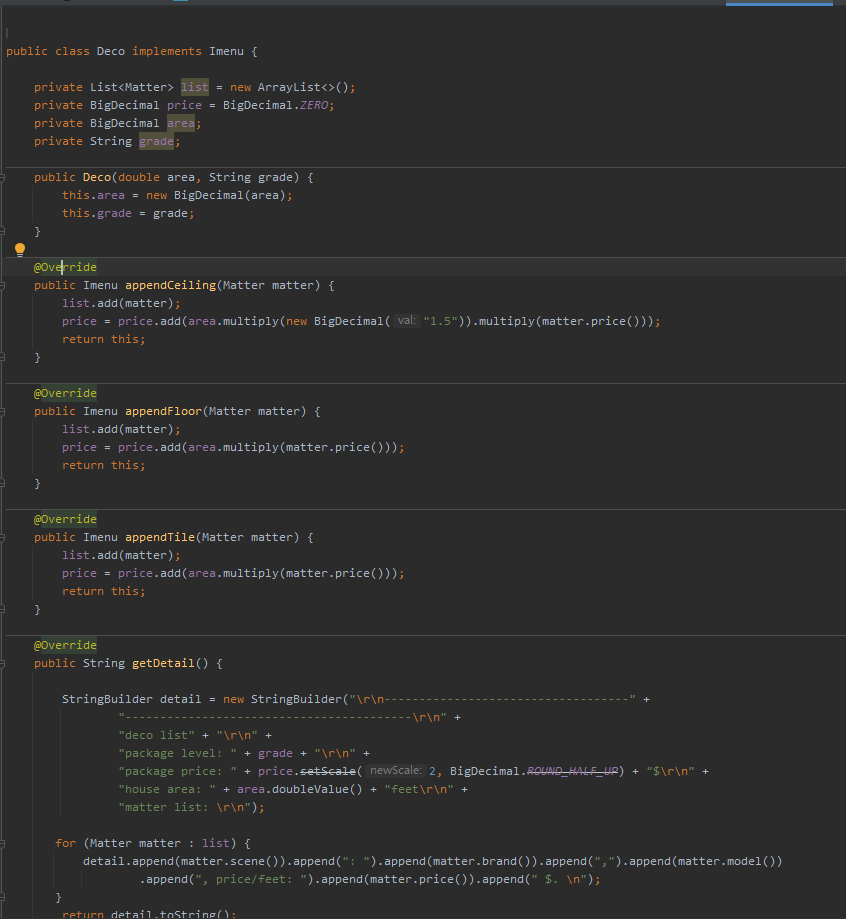
Builder
Builder
-
public Imenu packageOne (Double area) { return new Deco(area, “CLASSIC”) .appendCeiling(new LevelOneCeiling()) .appendFloor(new LevelOneFloor()) .appendTile(new LevelOneTile()); }
-
Imenu packageTwo (Double area) => new Deco(area, “MODERN”);
-
Imenu packageThree (Double area) => new Deco(area, “ART”);
2. Builder: design a fast food ordering system
1. food
// step 1 : Create an interface Item representing food item and packing.
-
Item (Interface) => name(), Packing packing(), price()
- Burger (abstract class) => Packing packing() => new Wrapper(), abstract float price()
- ChickenBurger
- VegBurger
- ColdDrink (abstract class) => Packing packing() => new Bottle(), abstract float price()
- Coke
- Pepsi
- Burger (abstract class) => Packing packing() => new Wrapper(), abstract float price()
2. packing
-
Packing (Interface) => pack()
- Bottle => pack() => “Bottle”
- Wrapper => pack() => “Wrapper”
Meal
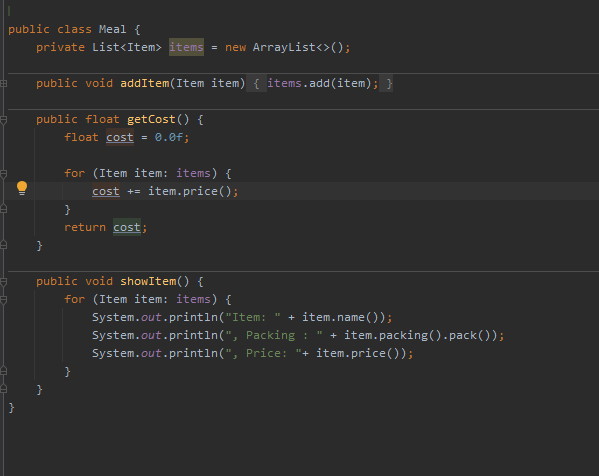
Builder
MealBuilder
- public Meal prepareMeal () {
Meal meal = new Meal();
meal.addItem(new VegBurger());
meal.addItem(new Coke());
return new meal; }
3. Builder: design a game actors system
Actor => type, sex, face, costume, hairstyle
abstract ActorBuilder
public Actor actor = new Actor();
public abstract void buildType();
public abstract void buildSex();
public abstract void buildFace();
public abstract void buildCostume();
public abstract void buildHairstyle();
public Actor createdActor() {
return actor;
}
}
AngleBuilder => extend abstract ActorBuilder => void buildType() => actor.setType(“Angle”);
DevilBuilder => extend abstract ActorBuilder
HeroBuilder => extend abstract ActorBuilder
ActorController
+ public **Actor** constructor(ActorBuilder ab) {
Actor actor
ab.buildType();
...
actor = ab.createActor();
return actor;
}
Client
- ActorBuilder actorBuilder = new HeroBuilder();
- ActorController actorController = new ActorController();
- Actor construct = actorController.construct(actorBuilder);
conbine ActorBuilder and ActorController
abstract ActorBuilder
protected static Actor actor = new Actor();
public abstract void buildType();
public abstract void buildSex();
public abstract void buildFace();
public abstract void buildCostume();
public abstract void buildHairstyle();
// public Actor createdActor() {
// return actor;
// }
// hooked method
public boolean isBareHeaded() {
return false;
}
public static Actor constructor(ActorBuilder ab) {
Actor actor
ab.buildType();
...
// hooked method
if (!ab.isBareHeaded()) {
ab.buildHairstyle();
}
return actor;
}
}
Client
- Actor construct = ActorBuilder.construct(new HeroBuilder());
1.Prototype pattern: design a WeeklyReport
In Prototype pattern, there are three roles
1. Prototype(parent for ConcretePrototype)
2. ConcretePrototype(implement the method in Protype parent, return a cloned object)
3. Client(objectify directly or use factory method to create a prototype object, invoke the clone method get multiple objects)
shallowClone
Attachment
public class Attachement {
private String name;
public void setName(String name) {
this.name = name;
}
public String getName() {
return this.name;
}
public void download() {
System.out.println("the file name is : " + name);
}
}
Log
public class Log implements Cloneable {
private String name;
private String date;
private String content;
// shallowClone
private Attachement attachement;
// shallowClone
public void setAttachement(Attachement attachement) {
this.attachement = attachement;
}
public String getName() {
return name;
}
// shallowClone
public Attachement getAttachement() {
return this.attachement;
}
public void setName(String name) {
this.name = name;
}
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
// Clone()
public Log Clone() t {
Object obj = null;
try {
obj = super.clone();
return (log)obj;
} catch(CloneNotSupportedException e) {
System.out.println("not support to chone this object: ");
return null;
}
}
}
deepClone
Attachment
Prototype
Log
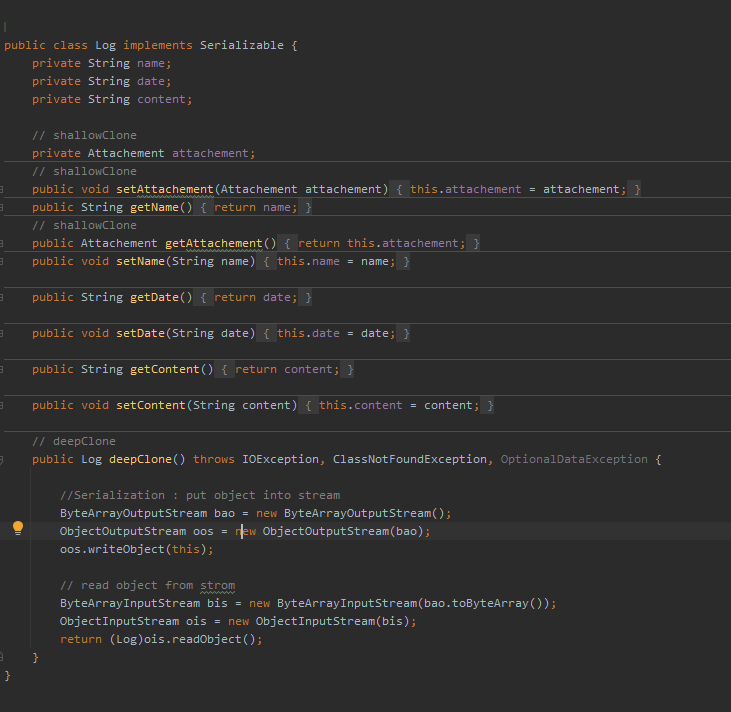
Prototype
//Serialization : put object into stream
ByteArrayOutputStream bao = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(bao);
oos.writeObject(this);
// read object from strom
ByteArrayInputStream bis = new ByteArrayInputStream(bao.toByteArray());
ObjectInputStream ois = new ObjectInputStream(bis);
return (Log)ois.readObject();
Prototype Manager :store prototype objects in a set, is a factory inchange of choning
OfficialDocument
interface OfficialDocument extends Cloneable
{
public OfficialDocument clone();
public void display();
}
Feasibility Analysis Report
class FAR implements OfficialDocument
{
public OfficialDocument clone()
{
OfficialDocument far = null;
try
{
far = (OfficialDocument)super.clone();
}
catch(CloneNotSupportedException e)
{
System.out.println("not support...!");
}
return far;
}
public void display()
{
System.out.println("Feasibility Analysis Report");
}
}
Software Requirements Specification
class SRS implements OfficialDocument
{
public OfficialDocument clone()
{
OfficialDocument srs = null;
try
{
srs = (OfficialDocument)super.clone();
}
catch(CloneNotSupportedException e)
{
System.out.println("not support...!");
}
return srs;
}
public void display()
{
System.out.println("Software Requirements Specification");
}
}
PrototypeManager
public class PrototypeManager {
private HashMap hashMap = new HashMap();
private static PrototypeManager pm = new PrototypeManager();
private PrototypeManager() {
hashMap.put("far", new FAR());
hashMap.put("far", new SRS());
}
public void addOfficialDocu(String key, OfficialDocu doc) {
hashMap.put(key, doc);
}
//shallowClone
public OfficialDocu getOffDocu(String key) {
return ((OfficialDocu) hashMap.get(key)).clone();
}
public static PrototypeManager getPrototypeManager() {
return pm;
}
Client
public class Client1 {
public static void main(String[] args) {
PrototypeManager pm = PrototypeManager.getPrototypeManager();
OfficialDocu doc1, doc2, doc3, doc4;
doc1 = pm.getOffDocu("far");
doc1.display();
doc2 = pm.getOffDocu("far");
doc2.display();
System.out.println(doc1 == doc2);
}
}
Prototype(OfficialDocument)
interface Prototype
{
public Prototype clone();
public void display();
}
PrototypeManager2
public class PrototypeManager {
private static Map<String, ProtoType> mProtos = new HashMap<>();
private PrototyManager() {
}
public static void setProtoType() {
}
public ProtoType getProto(Class<? extends ProtoType> type) {
if (mProtos.get(type.getClass().getSimpleName()) == null) {
try {
mProtos.put(type.getSimpleName(), type.newInstance());
} catch (InstantiationException | IllegalAccessException e) {
e.printStackTrace();
}
}
return mProtos.get(type.getSimpleName());
}
Prototype :design a testbank
before: AnswerQuest contains all the questions and answers in this class
ChoiceQuest contains all the questions and answers in this class
QuestBankController conbine answerQuest and choiceQuest in this class
after:
utils
- Topic
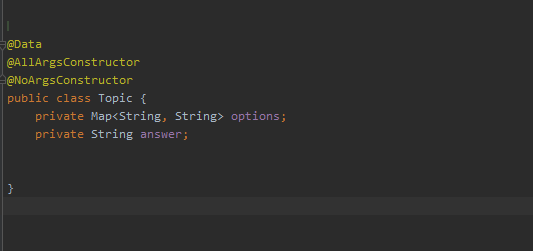
Prototype
- TopicRandom
public class TopicRandom {
static public Topic random(Map<String, String> option, String answer) {
Set<String> keySet = option.keySet();
List<String> keyList = new ArrayList<>(keySet);
Collections.shuffle(keyList);
HashMap<String, String> optionNew = new HashMap<>();
int index = 0;
String ansNew = "";
for (String next : keySet) {
String ranomKey = keyList.get(index++);
if (answer.equals(next)) {
ansNew = randomKey;
}
optionNew.put(randomKey, option.get(next));
}
return new Topic(optionNew, ansNew);
}
}
AnswerQuestion
Prototype
ChoiceQuestion
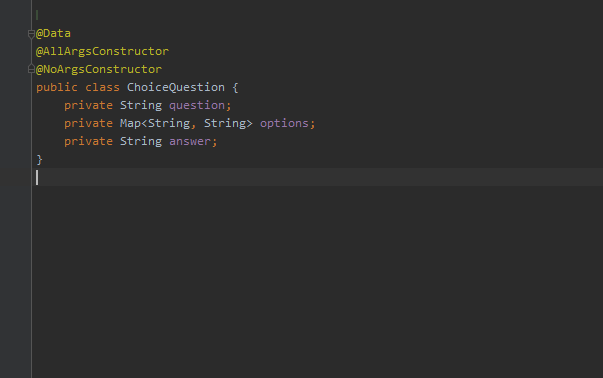
Prototype
ItemBank
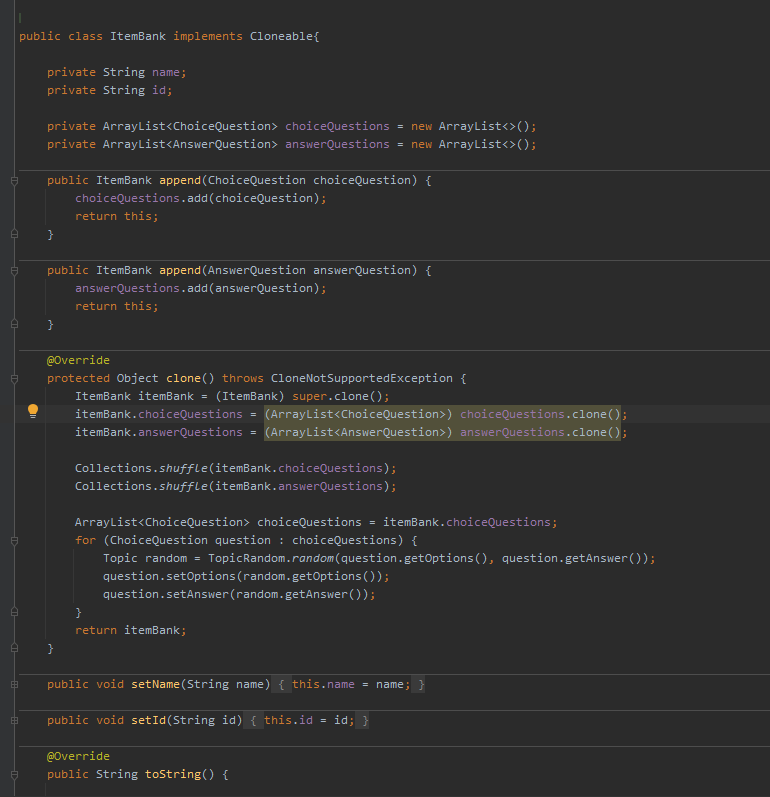
Prototype
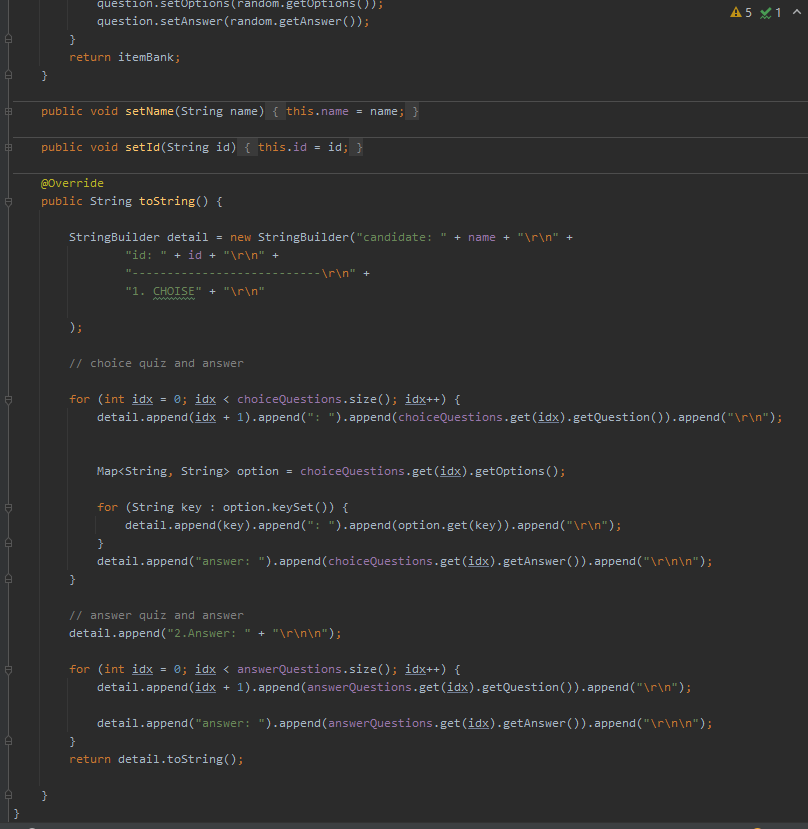
Prototype
BankController
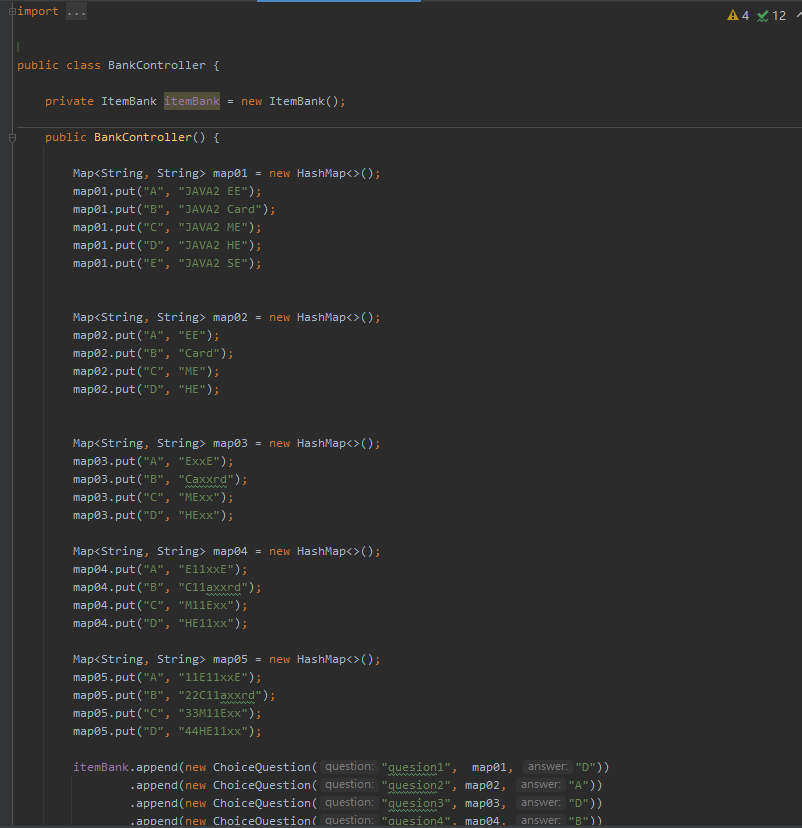
Prototype
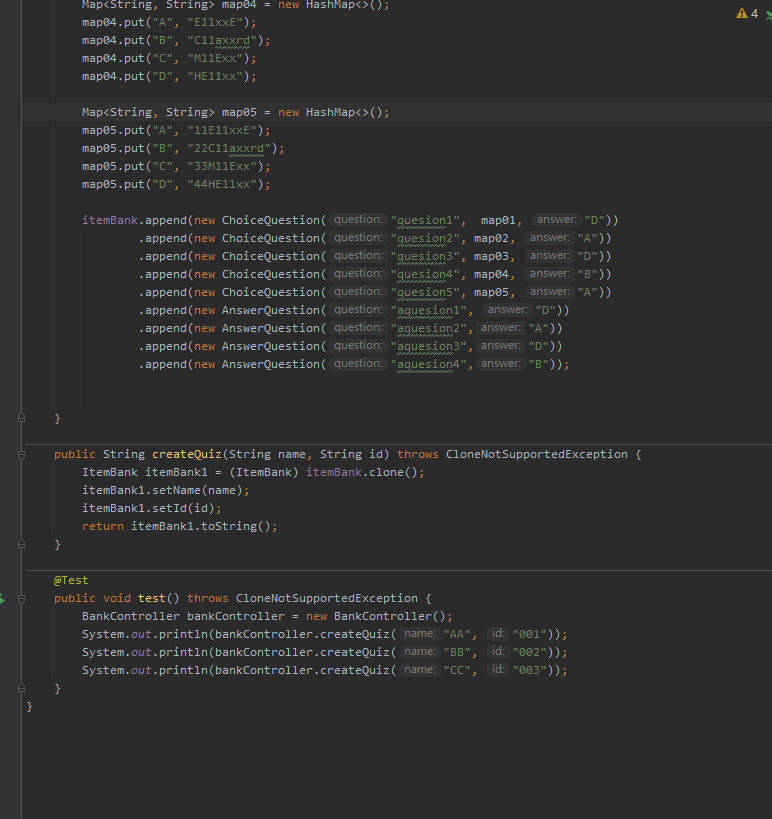
Prototype
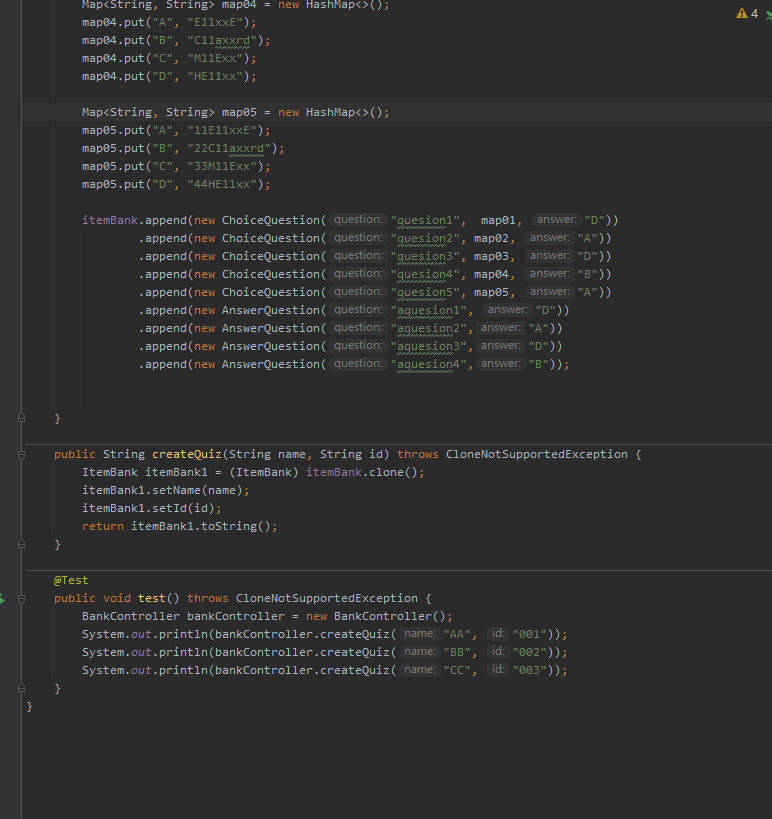